useCalendly()
The useCalendly()
composable is the easiest way to get started using Calendly. It provides a reactive object with all the methods provided by Calendly's widget.js.
Example
Check the Playground for an example of how the useCalendly()
composable works.
Methods
initBadgeWidget
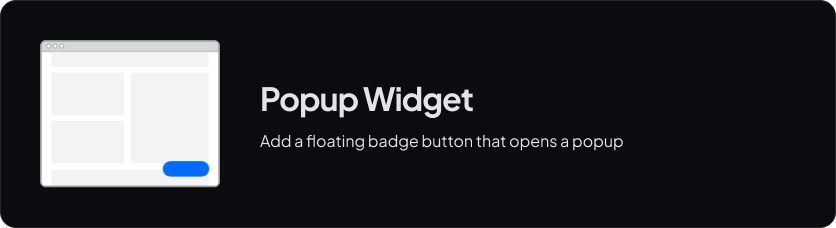
Use this method to display the "book a call" badge button on the bottom right of your page.
See <CalendlyPopupWidget />
Options for all available options.
<script lang="ts" setup>
const calendly = useCalendly()
// Loads the badge on page/component mount
onMounted(() => {
calendly.initBadgeWidget({
url: 'https://calendly.com/YOUR_LINK/30min',
})
})
</script>
initPopupWidget
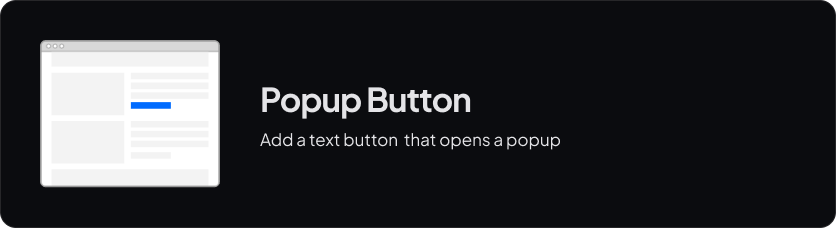
Use this method to open a fullscren modal popup on your page.
See Options for all available options.
<template>
<button @click="handleClick">Schedule time with me</button>
</template>
<script lang="ts" setup>
const calendly = useCalendly()
const handleClick = () => {
calendly.initPopupWidget({
url: 'https://calendly.com/YOUR_LINK/30min',
})
}
</script>
initInlineWidget
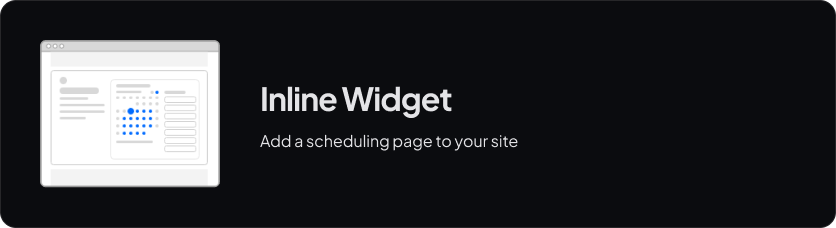
Use this method to embed the widget right into your page.
See <CalendlyInlineWidget />
Options for all available options.
<CalendlyInlineWidget />
component.Requirements
- The container div must have set a
min-width
andheight
attribute on it - It must have set the
calendly-inline-widget
class attribute on it - It must have set the url as a
data-url
attribute on it
<template>
<div
class="calendly-inline-widget"
data-url="https://calendly.com/YOUR_LINK/30min"
style="min-width: 320px; height: 630px;"
/>
</template>
<script lang="ts" setup>
const calendly = useCalendly()
onMounted(() => {
calendly.initInlineWidget()
})
</script>
showPopupWidget
Does the same then initPopupWidget
but without any configuration options other than the url
.
<template>
<button @click="handleClick">Schedule time with me</button>
</template>
<script lang="ts" setup>
const calendly = useCalendly()
const handleClick = () => {
calendly.showPopupWidget('https://calendly.com/YOUR_LINK/30min')
}
</script>
closePopupWidget
Closes the popup widget, which was opened before via initPopupWidget
or showPopupWidget
.
destroyBadgeWidget
Removes the badge widget, which was added before via initBadgeWidget
.